Credits: @dhy_pw
Thanks to: Pak Muhsin Shodiq
Menyambut ultah nyit-nyit.net yang ke-12, baru kali ini saya coba untuk share ke rekan" sekalian berupa tutorial, tutorial apa kk? kali ini kita bahas tentang pengenalan "Graphical User Interface" pada Java wkwkwk...
Subtopic nya apa aja kk?
Saya sudah download Eclipse Luna nih kk, selanjutnya gimana?
Ok...langsung saja kita praktekan, pada contoh kali ini saya akan membuat aplikasi registrasi ID (ingat hanya layout nya saja), pahami langkah-langkah berikut...
1. Import library, 3 ini penting...
2. Buat variabel yang kita butuhkan
3. Pembuatan Objek
4. Pengaturan Layout
Sekarang tinggal kita gabungkan langkah-langkah tersebut menjadi 1 kerangka...
Full Source Code
Selesai, sekarang kita lihat ouput yang dihasilkan...
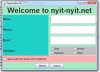
Saat ini sampai di Layout dulu...Next release akan saya lanjutkan dalam pembuatan fungsi di layout tersebut, dimana kita akan masuk ke tahap "Event Driven Programming"...
Like This yo...
Thanks to: Pak Muhsin Shodiq
Menyambut ultah nyit-nyit.net yang ke-12, baru kali ini saya coba untuk share ke rekan" sekalian berupa tutorial, tutorial apa kk? kali ini kita bahas tentang pengenalan "Graphical User Interface" pada Java wkwkwk...
Subtopic nya apa aja kk?
- Swing vs AWT
- The Java GUI API
- Frames
- Basic Layout Manager
- Panels
- Label, TextField and Button
- Run a simple Java GUI
- Dapat menerapkan konsep pemrograman berorientasi objek teknologi Java
- Menunjukkan pengguna grafis antarmuka pemrograman teknologi Java
Saya sudah download Eclipse Luna nih kk, selanjutnya gimana?
Ok...langsung saja kita praktekan, pada contoh kali ini saya akan membuat aplikasi registrasi ID (ingat hanya layout nya saja), pahami langkah-langkah berikut...
1. Import library, 3 ini penting...
Code:
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
Code:
private final Color RADIO_BUTTON_BG_COLOR = Color.LIGHT_GRAY;
private JLabel mainTitle;
private JTextField textName;
private JTextField textPhone;
private JTextField textEmail;
private JRadioButton radioGold;
private JRadioButton radioBronze;
private JRadioButton radioPlatinum;
private JRadioButton radioSilver;
private JCheckBox checkAggree;
private JButton btnSubmit;
private JButton btnCancel;
private JLabel nameLabel;
private JLabel phoneLabel;
private JLabel emailLabel;
private JLabel categoryLabel;
private ButtonGroup categoryGroup;
Code:
mainTitle = new JLabel("Welcome to nyit-nyit.net", JLabel.CENTER);
mainTitle.setFont(new Font("Arial", Font.BOLD, 40));
mainTitle.setBorder(BorderFactory.createEmptyBorder(0, 20, 0, 20));
textName = new JTextField();
textPhone = new JTextField();
textEmail = new JTextField();
radioGold = new JRadioButton("Gold");
radioGold.setBackground(RADIO_BUTTON_BG_COLOR);
radioBronze = new JRadioButton("Bronze");
radioBronze.setBackground(RADIO_BUTTON_BG_COLOR);
radioPlatinum = new JRadioButton("Platinum");
radioPlatinum.setBackground(RADIO_BUTTON_BG_COLOR);
radioSilver = new JRadioButton("Silver");
radioSilver.setBackground(RADIO_BUTTON_BG_COLOR);
checkAggree = new JCheckBox("I agree with this terms and conditions");
checkAggree.setBackground(Color.PINK);
btnSubmit = new JButton("Submit");
btnCancel = new JButton("Cancel");
Font fieldLabelFont = new Font("Arial", Font.BOLD, 15);
nameLabel = new JLabel("Name :");
nameLabel.setFont(fieldLabelFont);
phoneLabel = new JLabel("Phone :");
phoneLabel.setFont(fieldLabelFont);
emailLabel = new JLabel("Email :");
emailLabel.setFont(fieldLabelFont);
categoryLabel = new JLabel("Category :");
categoryLabel.setFont(fieldLabelFont);
categoryGroup = new ButtonGroup();
categoryGroup.add(radioGold);
categoryGroup.add(radioBronze);
categoryGroup.add(radioPlatinum);
categoryGroup.add(radioSilver);
Code:
JPanel radioPanel = new JPanel();
radioPanel.setLayout(new GridLayout(2,2));
radioPanel.add(radioGold);
radioPanel.add(radioBronze);
radioPanel.add(radioPlatinum);
radioPanel.add(radioSilver);
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout(FlowLayout.CENTER));
buttonPanel.setOpaque(false);
buttonPanel.add(btnSubmit);
buttonPanel.add(btnCancel);
JPanel firstColumnPanel = new JPanel();
firstColumnPanel.setLayout(new GridLayout(4,1,0,10));
firstColumnPanel.setBackground(new Color(29, 209, 200));
firstColumnPanel.add(nameLabel);
firstColumnPanel.add(phoneLabel);
firstColumnPanel.add(emailLabel);
firstColumnPanel.add(categoryLabel);
JPanel secondColumnPanel = new JPanel();
secondColumnPanel.setLayout(new GridLayout(4,1,0,10));
secondColumnPanel.setOpaque(false);
secondColumnPanel.add(textName);
secondColumnPanel.add(textPhone);
secondColumnPanel.add(textEmail);
secondColumnPanel.add(radioPanel);
JPanel centerPanel = new JPanel();
centerPanel.setLayout(new GridLayout(1,2,10,0));
centerPanel.setOpaque(false);
centerPanel.add(firstColumnPanel);
centerPanel.add(secondColumnPanel);
JPanel southPanel = new JPanel();
southPanel.setLayout(new GridLayout(2,1));
southPanel.setOpaque(false);
southPanel.add(checkAggree);
southPanel.add(buttonPanel);
JPanel mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
mainPanel.add(mainTitle, BorderLayout.NORTH);
mainPanel.add(centerPanel, BorderLayout.CENTER);
mainPanel.add(southPanel, BorderLayout.SOUTH);
mainPanel.setBackground(new Color(166,237,197));
this.add(mainPanel);
Sekarang tinggal kita gabungkan langkah-langkah tersebut menjadi 1 kerangka...
Full Source Code
Code:
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class GUI extends JFrame {
private final Color RADIO_BUTTON_BG_COLOR = Color.LIGHT_GRAY;
private JLabel mainTitle;
private JTextField textName;
private JTextField textPhone;
private JTextField textEmail;
private JRadioButton radioGold;
private JRadioButton radioBronze;
private JRadioButton radioPlatinum;
private JRadioButton radioSilver;
private JCheckBox checkAggree;
private JButton btnSubmit;
private JButton btnCancel;
private JLabel nameLabel;
private JLabel phoneLabel;
private JLabel emailLabel;
private JLabel categoryLabel;
private ButtonGroup categoryGroup;
public GUI() {
super("Registration ID");
initObject();
setFormLayout();
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private void initObject() {
mainTitle = new JLabel("Welcome to nyit-nyit.net", JLabel.CENTER);
mainTitle.setFont(new Font("Arial", Font.BOLD, 40));
mainTitle.setBorder(BorderFactory.createEmptyBorder(0, 20, 0, 20));
textName = new JTextField();
textPhone = new JTextField();
textEmail = new JTextField();
radioGold = new JRadioButton("Gold");
radioGold.setBackground(RADIO_BUTTON_BG_COLOR);
radioBronze = new JRadioButton("Bronze");
radioBronze.setBackground(RADIO_BUTTON_BG_COLOR);
radioPlatinum = new JRadioButton("Platinum");
radioPlatinum.setBackground(RADIO_BUTTON_BG_COLOR);
radioSilver = new JRadioButton("Silver");
radioSilver.setBackground(RADIO_BUTTON_BG_COLOR);
checkAggree = new JCheckBox("I agree with this terms and conditions");
checkAggree.setBackground(Color.PINK);
btnSubmit = new JButton("Submit");
btnCancel = new JButton("Cancel");
Font fieldLabelFont = new Font("Arial", Font.BOLD, 15);
nameLabel = new JLabel("Name :");
nameLabel.setFont(fieldLabelFont);
phoneLabel = new JLabel("Phone :");
phoneLabel.setFont(fieldLabelFont);
emailLabel = new JLabel("Email :");
emailLabel.setFont(fieldLabelFont);
categoryLabel = new JLabel("Category :");
categoryLabel.setFont(fieldLabelFont);
categoryGroup = new ButtonGroup();
categoryGroup.add(radioGold);
categoryGroup.add(radioBronze);
categoryGroup.add(radioPlatinum);
categoryGroup.add(radioSilver);
}
private void setFormLayout() {
JPanel radioPanel = new JPanel();
radioPanel.setLayout(new GridLayout(2,2));
radioPanel.add(radioGold);
radioPanel.add(radioBronze);
radioPanel.add(radioPlatinum);
radioPanel.add(radioSilver);
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout(FlowLayout.CENTER));
buttonPanel.setOpaque(false);
buttonPanel.add(btnSubmit);
buttonPanel.add(btnCancel);
JPanel firstColumnPanel = new JPanel();
firstColumnPanel.setLayout(new GridLayout(4,1,0,10));
firstColumnPanel.setBackground(new Color(29, 209, 200));
firstColumnPanel.add(nameLabel);
firstColumnPanel.add(phoneLabel);
firstColumnPanel.add(emailLabel);
firstColumnPanel.add(categoryLabel);
JPanel secondColumnPanel = new JPanel();
secondColumnPanel.setLayout(new GridLayout(4,1,0,10));
secondColumnPanel.setOpaque(false);
secondColumnPanel.add(textName);
secondColumnPanel.add(textPhone);
secondColumnPanel.add(textEmail);
secondColumnPanel.add(radioPanel);
JPanel centerPanel = new JPanel();
centerPanel.setLayout(new GridLayout(1,2,10,0));
centerPanel.setOpaque(false);
centerPanel.add(firstColumnPanel);
centerPanel.add(secondColumnPanel);
JPanel southPanel = new JPanel();
southPanel.setLayout(new GridLayout(2,1));
southPanel.setOpaque(false);
southPanel.add(checkAggree);
southPanel.add(buttonPanel);
JPanel mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
mainPanel.add(mainTitle, BorderLayout.NORTH);
mainPanel.add(centerPanel, BorderLayout.CENTER);
mainPanel.add(southPanel, BorderLayout.SOUTH);
mainPanel.setBackground(new Color(166,237,197));
this.add(mainPanel);
}
private void resetForm() {
textName.setText("");
textPhone.setText("");
textEmail.setText("");
categoryGroup.clearSelection();
checkAggree.setSelected(false);
}
public static void main(String[] args) {
GUI mainForm = new GUI();
mainForm.pack();
mainForm.setVisible(true);
}
}
Selesai, sekarang kita lihat ouput yang dihasilkan...
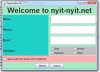
Saat ini sampai di Layout dulu...Next release akan saya lanjutkan dalam pembuatan fungsi di layout tersebut, dimana kita akan masuk ke tahap "Event Driven Programming"...
Like This yo...
Last edited: